Transforming Values
Draftbit comes with built-in transform utility functions to transform data on-the-fly. You can also use your own Functions to transform data.
If the value you are working with supports being transformed, the 'transform' dropdown will be displayed below it allowing you to apply one or more transforms to the value.
These functions work on components like Text and Set Variable Actions on Touchable or Button Components. Or, use them to conditionally display a component.
It's possible to chain multiple transforms together and use conditional statements to perform more complex manipulation of your value. This includes both the built-in transforms and your own transform functions.
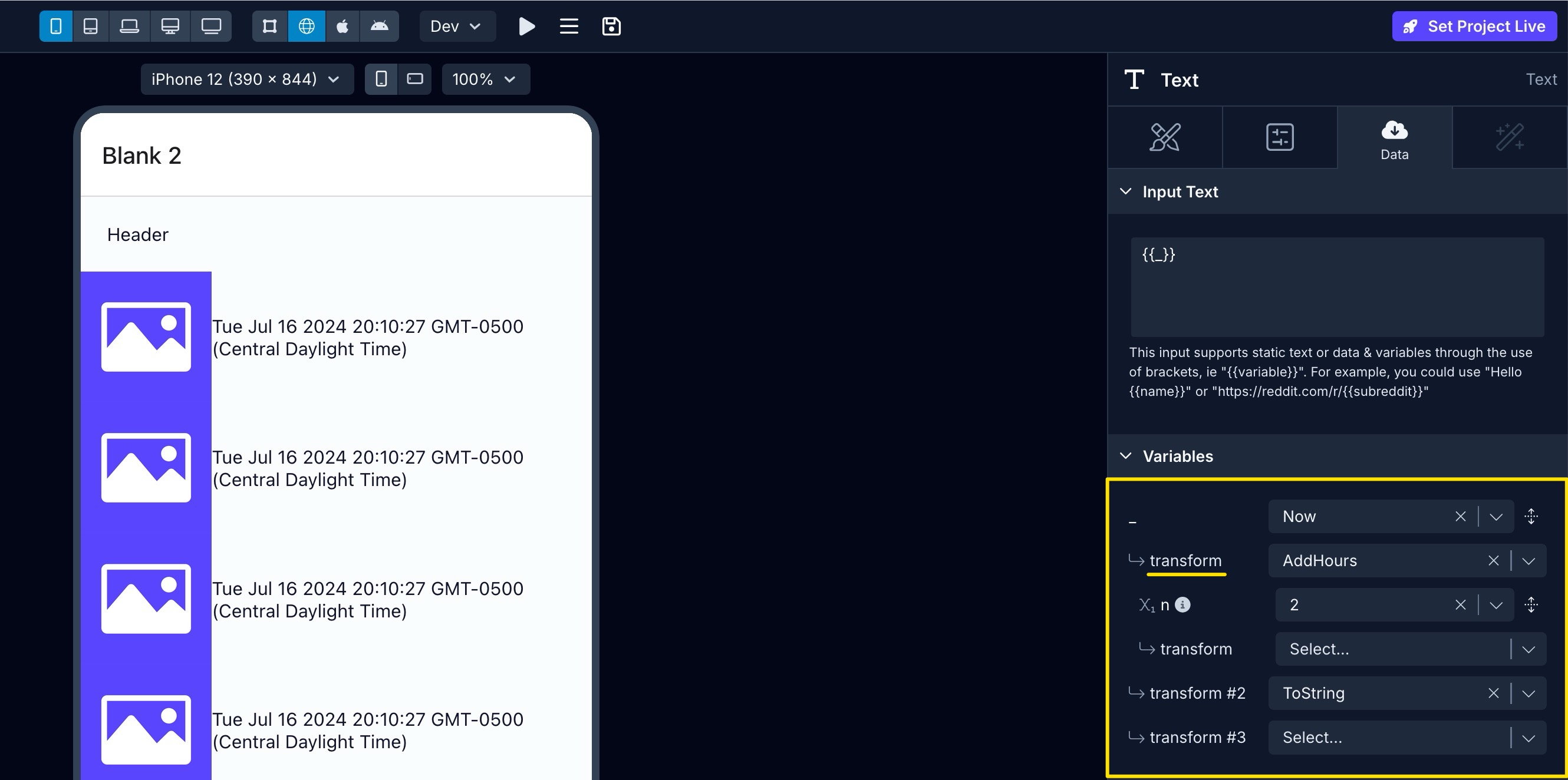
Keypath
If the value you are transforming is an object or array and you just want to access nested data, then you can type the keypath directly into the transform input to target specific indexes and keys.
In the case of an object, we can use simple dot notation (.
) to get the value of a specific key. Consider a value like this assigned to a Variable named user
:
{
id: 1,
name: 'Dave',
company: 'Draftbit',
address: {
street: '123 Draftbit Way',
city: 'Austin',
state: 'Texas'
}
}
After we select the user
variable from the dropdown, we can get just the id
value by entering .id
into the transform input. Notice the period (.
) prefix. In the generated code, this will create user.id
which references the value 1
in the example object above.
Or, we could get the state
value by entering .address.state
which would generate user.address.state
which references the value Texas
.
In the case of an array, we can add the use of square brackets ([]
) to select specific indexes. Consider a value like this assigned to a Variable named teams
:
[
{
id: 1,
name: 'Development',
tags: ['engineering','software','coding']
},
{
id: 2,
name: 'Design',
tags: ['ui','ux','branding']
}
]
We can get just the name of the first item in the array by entering [0].name
where 0
is the first index in the array. The generated code would be teams[0].name
.
If we wanted the second tag in the second item in the array, we would enter [1].tags[1]
.
Date
Name | Description |
---|---|
AddSeconds | Adds the provided value in seconds to a Date object |
AddMinutes | Adds the provided value in minutes to a Date object |
AddHours | Adds the provided value in hours to a Date object |
AddDays | Adds the provided value in days to a Date object |
AddWeeks | Adds the provided value in weeks to a Date object |
AddMonths | Adds the provided value in months to a Date object |
AddYears | Adds the provided value in years to a Date object |
SubtractSeconds | Subtracts the provided value in seconds from a Date object |
SubtractMinutes | Subtracts the provided value in minutes from a Date object |
SubtractHours | Subtracts the provided value in hours from a Date object |
SubtractDays | Subtracts the provided value in days from a Date object |
SubtractWeeks | Subtracts the provided value in weeks from a Date object |
SubtractMonths | Subtracts the provided value in months from a Date object |
SubtractYears | Subtracts the provided value in years from a Date object |
FormatDate | Formats a Date object as a string using the provided format. For example, yyyy-MM-dd hh:mm:ss . See here for more. |
FormatDistanceToNow | Formats a Date object as a human-readable string relative to now. For example, less than a minute , about 1 year , etc. |
ParseDate | Parses a string representation of a date, and returns a Date object |
FormatDateISO | Converts a date object to an ISO-formatted date string. The standard is called ISO-8601 and the format is: YYYY-MM-DDTHH:mm:ss.sssZg |
String
Name | Description | Before | After |
---|---|---|---|
ParseBoolean | Function that takes a string value. If it's |
|
|
ParseInt | Parses a string and returns an integer. |
|
|
ParseFloat | Parses a string and returns a floating-point number. |
|
|
ParseJsonString | A function that converts a JSON string to a JavaScript object. |
|
|
AddBearerPrefix | A function returns the token with the prefix |
|
|
ToUpperCase | A function that converts a string to uppercase letters. |
|
|
ToLowerCase | A function that converts a string to lowercase letters. |
|
|
StringConcat | Concatenates the given strings |
|
|
Trim | Trims the whitespace from the start and end of the given string |
|
|
TrimStart | Trims the whitespace from the start of the given string |
|
|
TrimEnd | Trims the whitespace from the end of the given string |
|
|
StartsWith | Returns |
|
|
EndsWith | Returns |
|
|
Logic
Name | Description |
---|---|
If/Else | Used to further conditionally customize the chain of transforms |
And | Used to further conditionally customize the chain of transforms |
Or | Used to further conditionally customize the chain of transforms |
Negate | Function that takes a truthy value and changes it to falsy and vice versa. |
Numeric
Name | Description | Before | After |
---|---|---|---|
RoundUp | Rounds a numerical value up to the next largest integer. | 29.99 | 30 |
RoundDown | Rounds a numerical value down to the largest integer less than or equal to a given number. | 29.99 | 29 |
Increment | A function that increments the number by one. | 1 | 2 |
Decrement | A function that decrements the number by one. | 1 | 0 |
Numeric Negate | Returns the negated value of the provided value | 33 | -33 |
Add | The addition operator (+) adds numbers | 1 +2 | 3 |
Subtract | The subtraction operator (-) subtracts numbers | 3 - 2 | 1 |
Multiply | The multiplication operator (*) multiplies numbers. | 3 * 3 | 9 |
Divide | The division operator (/) divides numbers. | 99 / 3 | 33 |
Exponent | The exponentiation operator (**) raises the first operand to the power of the second operand. | 3 ** 2 | 9 |
Remainder (Modulus) | The modulus operator (%) returns the division remainder. | 33 % 3 | 0 |
Generic
Name | Description | Before | After |
---|---|---|---|
Length | Calculates the number of elements in an array or characters in a string and returns as a number |
|
|
JsonStringify | A function that converts a JavaScript object or value to a JSON string. |
|
|
ToString | A function that converts a given value to a string. |
|
|
Includes | Returns |
|
|
Slice | Returns the extracted part of a given string based on the provided start and end as a new string |
|
|
ConsoleLog | Prints the given value to the Builder's Console Logs |
Comparison
Name | Description | Before | After |
---|---|---|---|
EqualTo | A function that checks if two values are equal. | 1 and 1 or "foo" and "bar" | Returns true or false |
NotEqualTo | A function that checks if two values are unequal. | 1 and 1 or "foo" and "bar" | Returns false or true |
LessThan | A function that checks if the first value is less than the second value. | 1 < 10 | Returns true |
GreaterThan | A function that checks if the first value is greater than the second value. | 1 > 10 | Returns false |
LessThanOrEqual | A function that checks if the first value is less than or equal to the second value. | 1 <= 10 | Returns true |
GreaterThanOrEqual | A function that checks if the first value is greater than or equal to the second value. | 1 >= 10 | Returns false |
Array
Name | Description | Before | After |
---|---|---|---|
ArrayConcat | A function that transform an array but adding the items from a second array to the end of the first array. |
|
|
ArrayAppend | A Function that transform an array by adding the given value as the last item in the array. |
|
|
ArrayPrepend | A Function that transform an array by adding the given value as the first item in the array. |
|
|
ArrayLast | Returns the last item in the given array |
|
|
Custom Transforms
You can also use any Functions that have one or more parameters as a Transform Function by selecting it from the dropdown.
Multiple ArgumentsIf your function accepts more than one argument, you will have additional inputs to pass those values. The first value selected will always be passed as the first argument to the function.
Updated 7 months ago