Variables in Code
It's possible to use an App Variable, Device Variable, or Screen Variable with Custom Code.
Access in Custom Files
You can get and set App and Device Variables in Custom Files.
To access an App or Device Variable the initial step is to import the GlobalVariableContext
to access the module. You cannot use the GlobalVariableContext API without importing
If your file is located at the Top Level location (/
), import using
import * as GlobalVariableContext from './config/GlobalVariableContext';
Otherwise if it's in the Custom Files location (/custom-files
) directory, use
import * as GlobalVariableContext from '../config/GlobalVariableContext';
Reading a value with useValues
useValues
is a function that returns an object containing current values of App Variables and Device Variables in your App.
Here is an example snippet on how you can read the value of an App or a Device Variable:
import React from "react";
import { Text } from "react-native";
import * as GlobalVariableContext from "../config/GlobalVariableContext";
export const MyVariableDisplay = () => {
const variables = GlobalVariableContext.useValues();
return <Text> { variables.myGlobal } </Text>
};
Updating a value with useSetValue
useSetValue
is a function that returns a setter function that, when called with an object containing key
and value
fields, will set an App Variable or a Device Variable with the specified key to the specified value.
import React from 'react';
import { Button } from 'react-native';
import * as GlobalVariableContext from '../config/GlobalVariableContext';
export const MyComponent = () => {
const setGlobalVariable = GlobalVariableContext.useSetValue();
// RANDOM_VARIABLE is defined as an App Variable
return (
<Button
onPress={() => setGlobalVariable({ key: 'RANDOM_VARIABLE', value: 'new value' })}
title="Update a Variable"
/>
);
};
Access in Functions
Global Variables
You can read and write the value of an App or a Device Variable in Global and Screen Functions
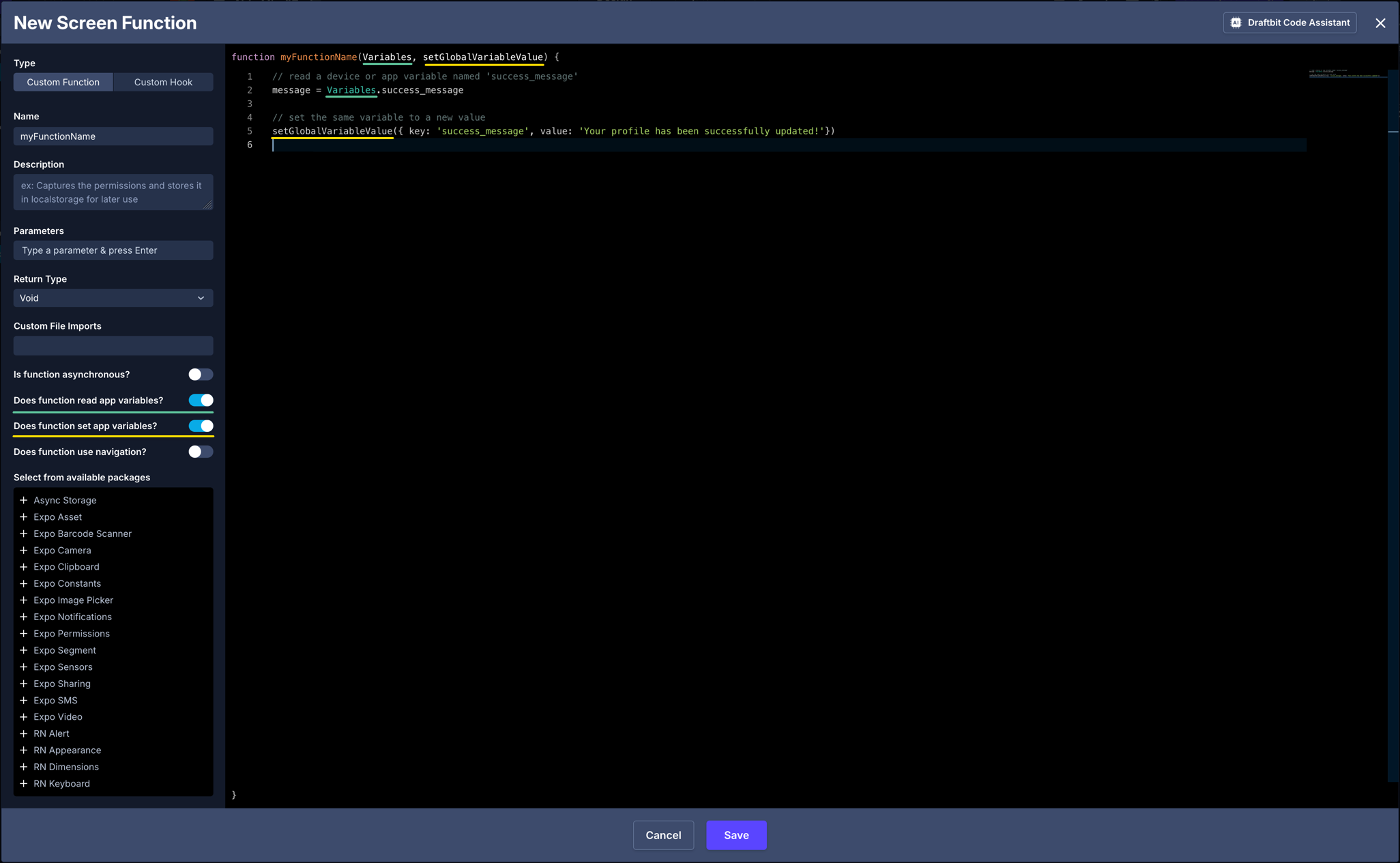
Inside your Function toggle on the option for Does function read app variables?. This will add a Variables
parameter to the function. In the example below, we have a variable named 'success_message' which you can then access like so:
// read a device or app variable named 'success_message'
message = Variables.success_message
You can also set a global variable by toggling on the option for Does function set app variables?. This will add a setGlobalVariableValue
parameter to your function. This property is itself a function that can then be used to set the value of a variable.
// set the 'success_message' app or device variable to a new value
setGlobalVariableValue({
key: 'success_message',
value: 'Your profile has been successfully updated!'
})
Screen Variables
You can read and write the value of a Screen Variable in Screen Functions.
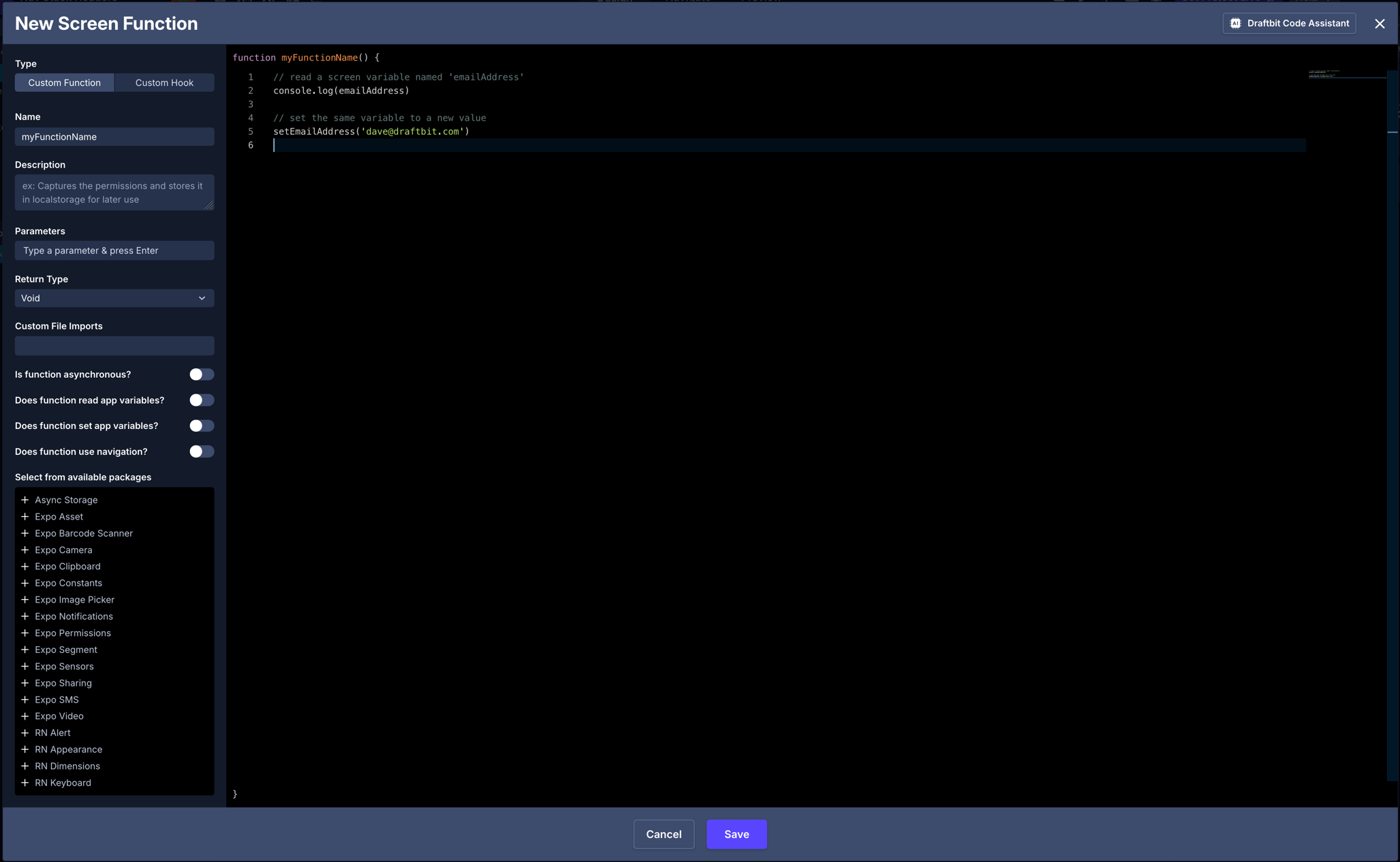
Since both Screen Variables and Screen Functions are in the same scope, no extra setup is required to start reading and writing Screen Variables inside of Screen Functions.
When you create a Screen Variable in Draftbit, you are actually creating state in React Native. For example, when you create a Screen Variable named emailAddress
we don't just create a variable like this:
let emailAddress = '[email protected]`
Instead we create a component state like this
const [emailAddress, setEmailAddress] = useState('[email protected]`)
As you can see, we've created a 'getter' and 'setter' for our state. Screen Variable setters will always be prefixed with set
.
If your variable is named
message
, then the getter will bemessage
and the setter will besetMessage
. Notice that camel case is used. If your variable is namedsuccess_message
, the setter will besetSuccess_message
.
So using the example above, in a Screen Function, we can access these directly like so:
console.log(emailAddress) //prints '[email protected]'
alert(emailAddress) //alerts '[email protected]'
Or we can update the value by using the setter
setEmailAddress('[email protected]')
Updated 7 months ago