Sending Push Notifications
With Expo's Push API
You can send push notifications using Expo's Push API from your backend or server.
Endpoint
https://exp.host/--/api/v2/push/send
Message Payload
{
"to": "ExponentPushToken[epJUA-PWB...]",
"title": "Hey",
"body": "There you go"
}
You can read more about the message payload in the Expo docs
Response
A successful response will return a Receipt ID which you can use to check the status of the notification
{
"data": {
"status": "ok",
"id": "327fe95c-9f00-44f5-a0be-2519dec0a3c0"
}
}
You can read more about the push ticket format in the Expo docs
From your app
Create a function to schedule the notification
- Open the Custom Code modal
- Add a new Function
- Name, enter schedulePushNotification
- Parameters, add expoPushToken, title, body
- Return Type, select String
- Enable Is function asynchronous?
- Under Select from available packages, click on Expo Notifications
- Add the following code
let receiptID = await Notifications.scheduleNotificationAsync({
content: {
to: expoPushToken,
title: title,
body: body
},
trigger: { seconds: 2 }
});
return receiptID;
Take a look at the docs for Expo Notifications for more info
The function will return a Receipt ID which you can use to get the status of the message. For more, take a look at the Expo docs
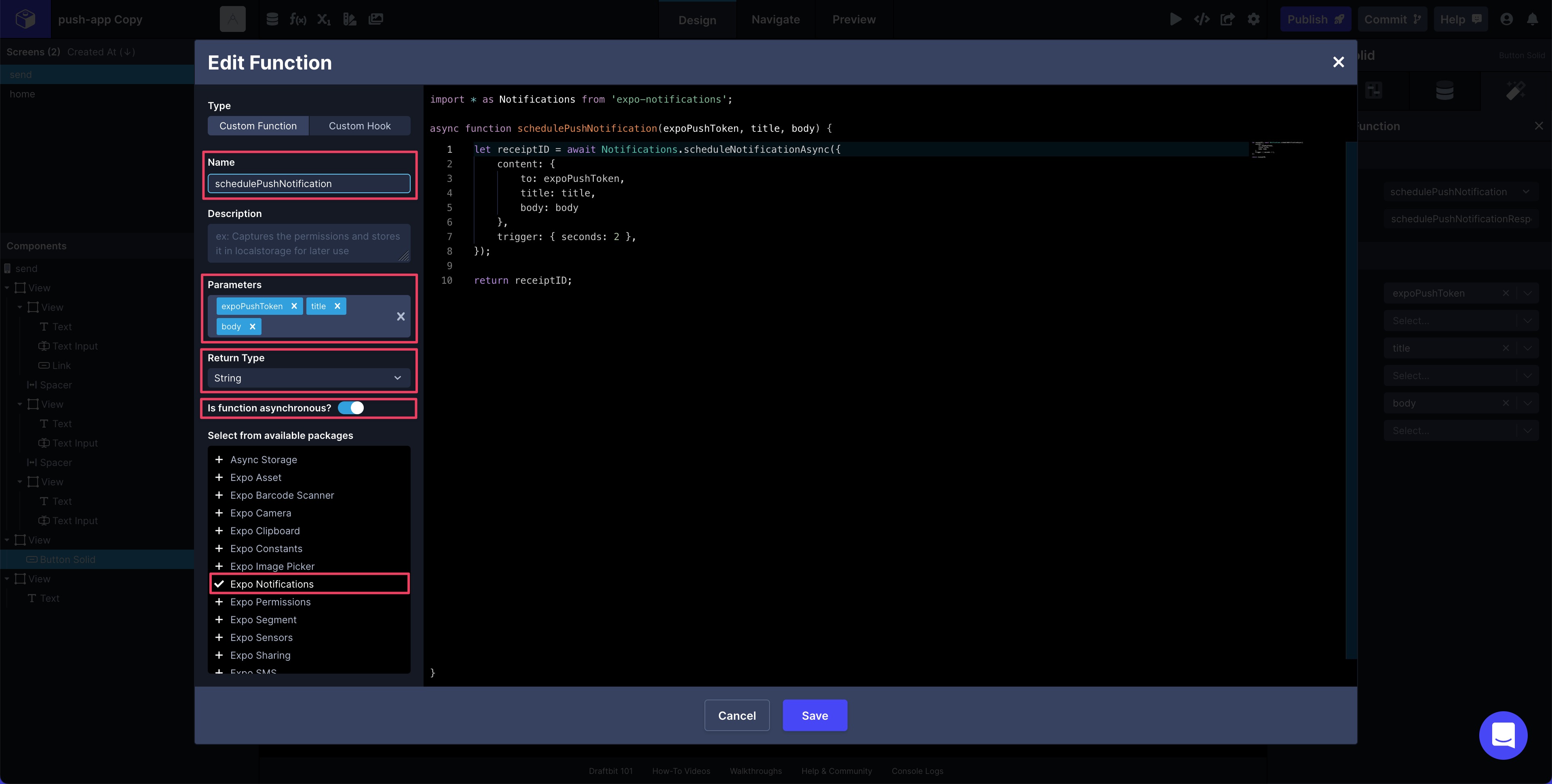
Trigger the function with an Action
You can use the Run a Custom Function Action in an Action Stack to trigger the schedulePushNotification Custom Function.
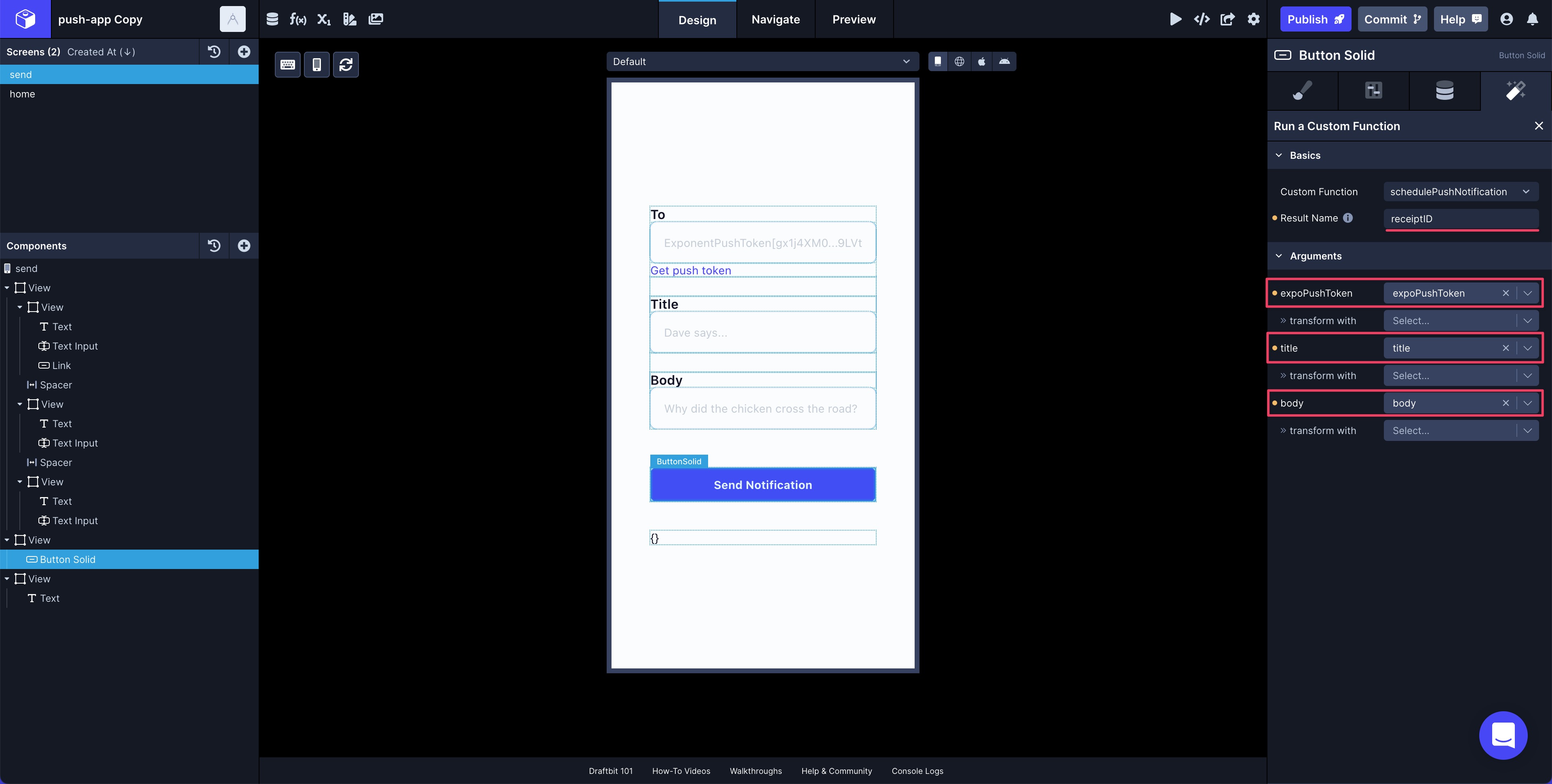
You can get a push token for your device to use for testing by using the Get Expo Push Token Action
From your server
There are also many SDK's available for various languages that allow you to interact with Expo's Push API from your server.
For more on Expo's Push SDKs, check out the docs here.
From your backend
Many BaaS services, like Xano, allow you to make API calls from your backend.
For more on sending notifications using Expo's Push REST API, check out the docs here
With Expo's Push Notification Tool
Expo provides a web-based Push Notification Tool you can use during development for testing purposes. You can check it out here.
Updated 5 months ago